realistic drawings of 3d objects
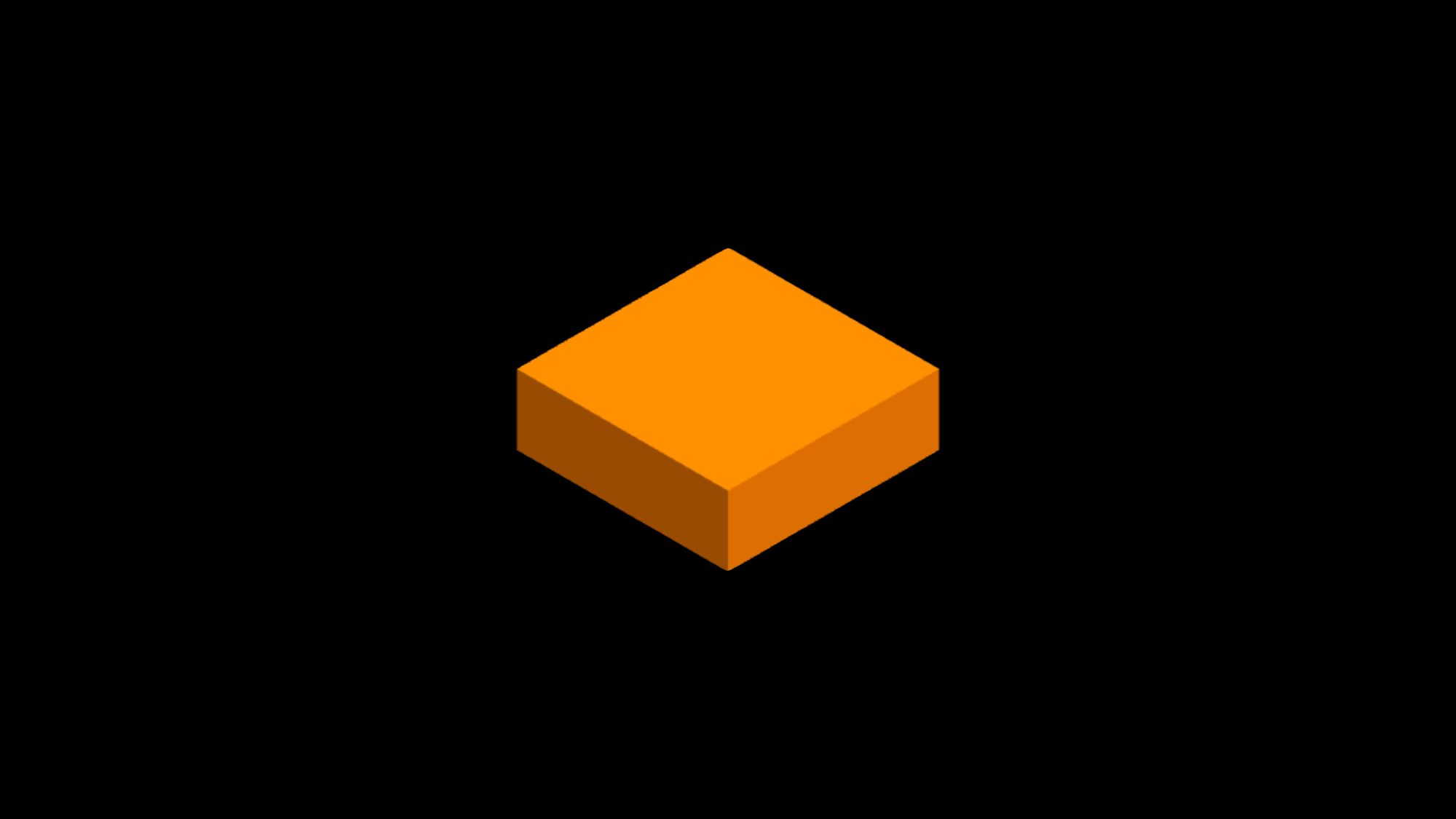
If you take ever wanted to build a game with JavaScript, you might have come across Three.js.
3.js is a library that we tin utilise to return 3D graphics in the browser. The whole thing is in JavaScript, and so with some logic you can add together animation, interaction, or even plough it into a game.
In this tutorial, we will get through a very uncomplicated example. We'll render a 3D box, and while doing so we'll larn the fundamentals of Iii.js.
Three.js uses WebGL under the hood to render 3D graphics. Nosotros could use plain WebGL, but information technology'southward very complex and rather low level. On the other mitt, Iii.js is like playing with Legos.
In this commodity, we'll get through how to place a 3D object in a scene, ready the lighting and a camera, and render the scene on a sail. So allow's run into how nosotros can exercise all this.
Define the Scene Object
First, we take to define a scene. This will be a container where nosotros identify our 3D objects and lights. The scene object also has some properties, like the background colour. Setting that is optional though. If we don't ready it, the default will be black.
import * every bit THREE from "three"; const scene = new THREE.Scene(); scene.background = new Three.Color(0x000000); // Optional, blackness is default ...
Geometry + Textile = Mesh
Then we add together our 3D box to the scene as a mesh. A mesh is a combination of a geometry and a fabric.
... // Add a cube to the scene const geometry = new THREE.BoxGeometry(3, 1, iii); // width, acme, depth const material = new THREE.MeshLambertMaterial({ colour: 0xfb8e00 }); const mesh = new THREE.Mesh(geometry, material); mesh.position.gear up(0, 0, 0); // Optional, 0,0,0 is the default scene.add(mesh); ...
What is a Geometry?
A geometry is a rendered shape that we're building - like a box. A geometry tin be build from vertices or we tin can employ a predefined one.
The BoxGeometry is the well-nigh bones predefined choice. Nosotros merely have to fix the width, height, and depth of the box and that'south it.
You might recollect that we can't get far by defining boxes, but many games with minimalistic design apply merely a combination of boxes.
In that location are other predefined geometries likewise. We can easily define a plane, a cylinder, a sphere, or fifty-fifty an icosahedron.
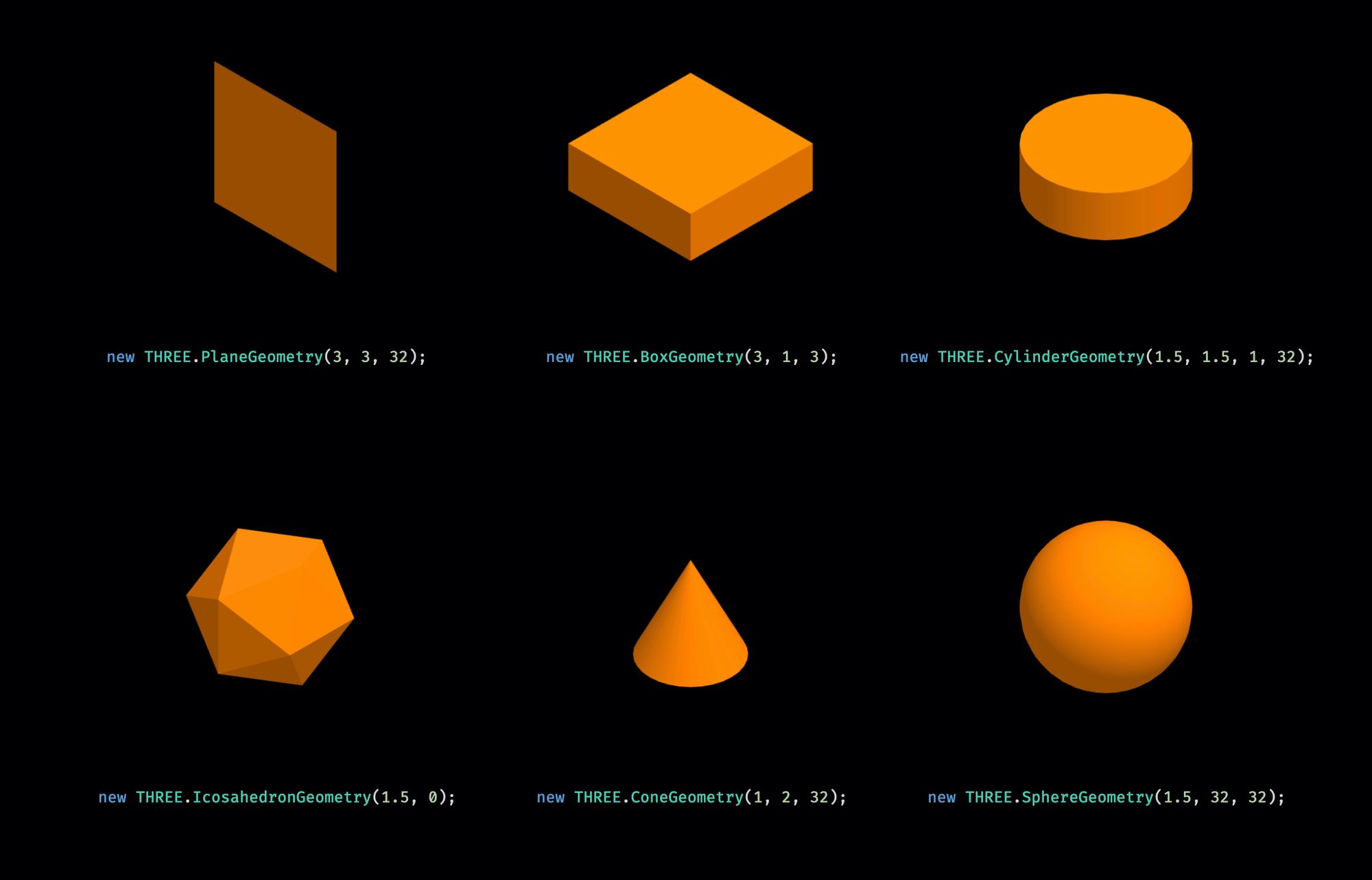
How to Piece of work with Cloth
Then we define a material. A material describes the advent of an object. Hither we tin define things like texture, color, or opacity.
In this case we are only going to set up a colour. There are all the same unlike options for materials. The primary difference between nearly of them is how they react to light.
The simplest one is the MeshBasicMaterial. This material doesn't care well-nigh light at all, and each side will take the same color. It might non exist the all-time option, though, as you can't see the edges of the box.
The simplest cloth that cares about light is the MeshLambertMaterial. This will calculate the color of each vertex, which is practically each side. But it doesn't become beyond that.
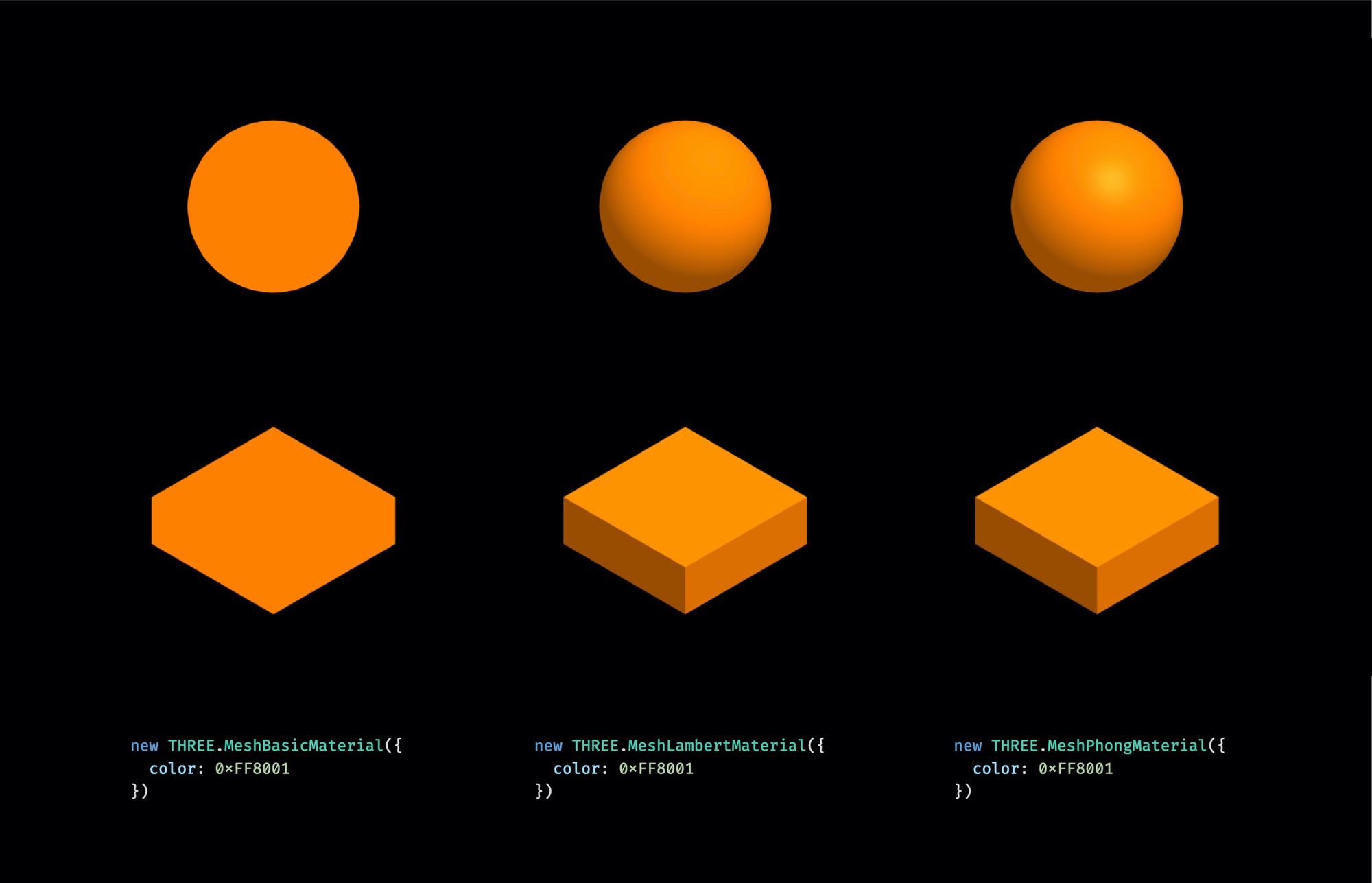
If you need more precision, there are more advanced materials. The MeshPhongMaterial not only calculates the colour by vertex only by each pixel. The colour tin change within a side. This can help with realism just also costs in operation.
It also depends on the low-cal settings and the geometry if information technology has whatsoever real consequence. If we render boxes and use directional calorie-free, the result won't modify that much. But if we render a sphere, the difference is more than obvious.
How to Position a Mesh
One time we accept a mesh we tin can also position it within the scene and ready a rotation past each axis. Subsequently if we desire to animate objects in the 3D space we volition mostly adjust these values.
For positioning we utilise the same units that we used for setting the size. It doesn't matter if y'all are using small numbers or large numbers, y'all simply need to be consistent in your own world.
For the rotation nosotros set the values in radians. So if y'all have your values in degrees yous have to carve up them by 180° and then multiply by PI.

How to Add Lite
Then permit'south add lights. A mesh with basic textile doesn't demand any light, as the mesh will have the set color regardless of the calorie-free settings.
But the Lambert material and Phong textile require light. If there isn't any light, the mesh will remain in darkness.
... // Prepare up lights const ambientLight = new Iii.AmbientLight(0xffffff, 0.6); scene.add(ambientLight); ...
We'll add 2 lights - an ambient light and a directional low-cal.
First, we add the ambient light. The ambient light is shining from every direction, giving a base of operations color for our geometry.
To set an ambient light we set a color and an intensity. The color is usually white, but you can fix whatsoever color. The intensity is a number between 0 and 1. The two lights we ascertain work in an accumulative fashion so in this case we want the intensity to be around 0.5 for each.

The directional calorie-free has a like setup, only it also has a position. The word position here is a chip misleading, because it doesn't hateful that the light is coming from an exact position.
The directional light is shining from very far abroad with many parallel light rays all having a fixed bending. But instead of defining angles, nosotros ascertain the direction of a unmarried light ray.
In this instance, information technology shines from the direction of the 10,20,0 position towards the 0,0,0 coordinate. But of course, the directional light is not only one low-cal ray, merely an infinite corporeality of parallel rays.
Think of it as the sun. On a smaller scale, light rays of the sun also come downwards in parallel, and the lord's day'southward position isn't what matters simply rather its direction.
And that's what the directional light is doing. It shines on everything with parallel calorie-free rays from very far away.
... const dirLight = new THREE.DirectionalLight(0xffffff, 0.6); dirLight.position.set(10, 20, 0); // ten, y, z scene.add(dirLight); ...
Hither we set the position of the low-cal to be from above (with the Y value) and shift it a bit along the 10-axis also. The Y-axis has the highest value. This means that the top of the box receives the most light and information technology will be the shiniest side of the box.
The lite is as well moved a bit forth the 10-axis, so the right side of the box will also receive some light, but less.
And because nosotros don't movement the light position forth the Z-axis, the front side of the box will not receive whatsoever light from this source. If at that place wasn't an ambience light, the front side would remain in darkness.
There are other light types as well. The PointLight, for example, can be used to simulate light bulbs. It has a stock-still position and information technology emits low-cal in every direction. And the SpotLight tin be used to simulate the spotlight of a auto. Information technology emits light from a single point into a direction along a cone.
How to Set up the Camera
So far, we have created a mesh with geometry and material. And we have as well ready up lights and added to the scene. We nonetheless need a photographic camera to ascertain how we look at this scene.
There are two options here: perspective cameras and orthographic cameras.
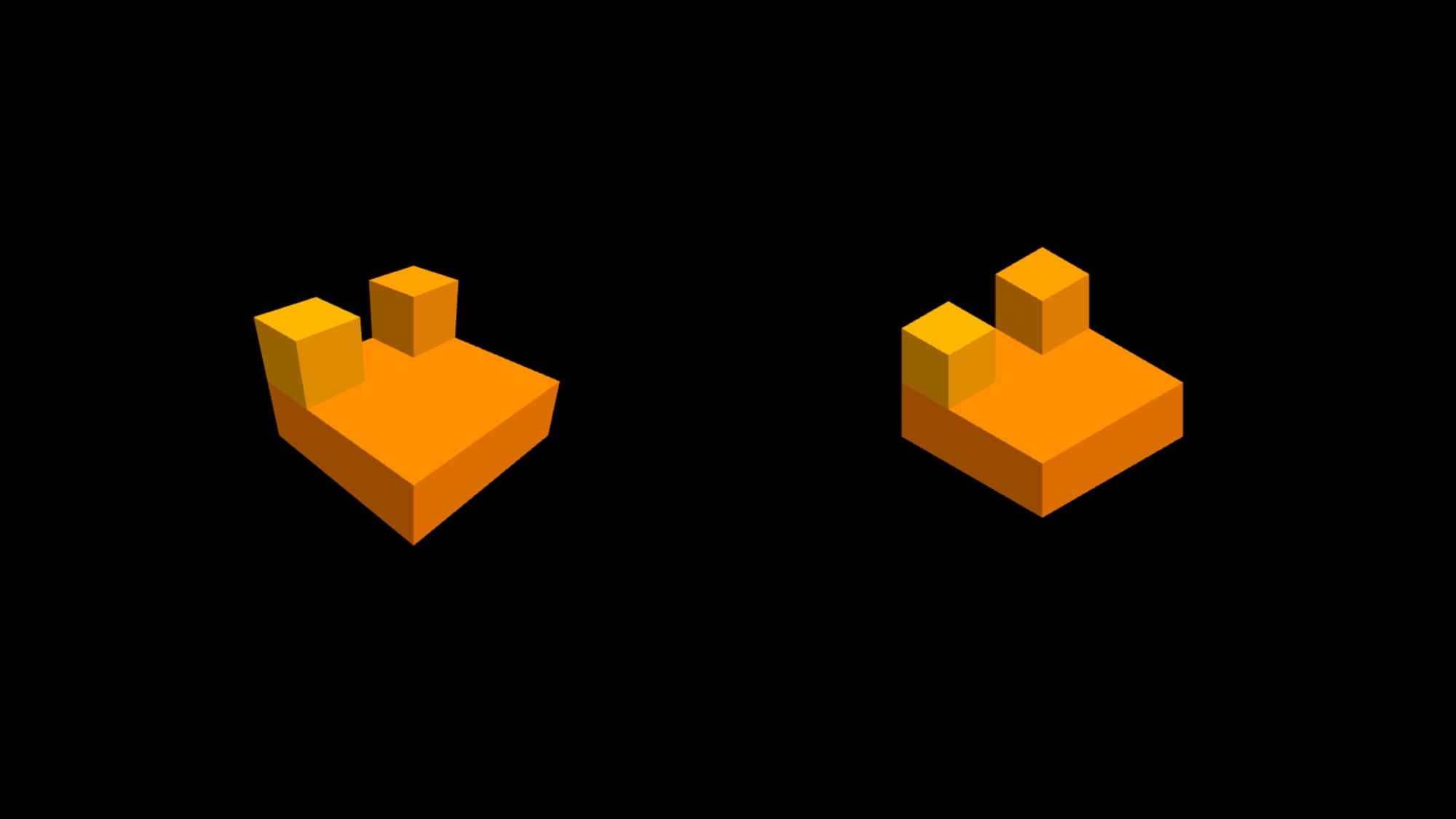
Video games more often than not utilize perspective cameras, because how they work is similar to how you lot see things in existent life. Things that are further away appear to be smaller and things that are correct in front of yous appear bigger.
With orthographic projections, things volition take the same size no matter how far they are from the camera. Orthographic cameras have a more minimal, geometric look. They don't distort the geometries - the parallel lines will appear in parallel.
For both cameras, we take to define a view frustum. This is the region in the 3D space that is going to exist projected to the screen. Anything outside of this region won't appear on the screen. This is considering it is either too close or likewise far away, or because the camera isn't pointed towards information technology.

With perspective projection, everything within the view frustum is projected towards the viewpoint with a directly line. Things further abroad from the camera appear smaller on the screen, because from the viewpoint you lot can see them nether a smaller angle.
... // Perspective photographic camera const attribute = window.innerWidth / window.innerHeight; const photographic camera = new THREE.PerspectiveCamera( 45, // field of view in degrees aspect, // aspect ratio one, // near plane 100 // far plane ); ...
To define a perspective photographic camera, you lot need to set a field of view, which is the vertical angle from the viewpoint. So you define an attribute ratio of the width and the height of the frame. If you fill the whole browser window and y'all want to keep its aspect ratio, then this is how you can do it.
Then the last two parameters define how far the near and far planes are from the viewpoint. Things that are besides shut to the camera volition be ignored, and things that are too far away will be ignored besides.
... // Orthographic camera const width = 10; const summit = width * (window.innerHeight / window.innerWidth); const camera = new THREE.OrthographicCamera( width / -two, // left width / 2, // right height / 2, // top acme / -two, // bottom 1, // nearly 100 // far ); ...
So at that place's the orthographic camera. Hither nosotros are non projecting things towards a single betoken simply towards a surface. Each projection line is in parallel. That'southward why it doesn't thing how far objects are from the camera, and that'southward why it doesn't distort geometries.
For orthographic cameras, we accept to define how far each plane is from the viewpoint. The left aeroplane is therefor five units to the left, and the right aeroplane is v units to the right, and so on.
... camera.position.gear up(4, iv, 4); camera.lookAt(0, 0, 0); ...
Regardless of which camera are we using, we also demand to position it and gear up it in a direction. If we are using an orthographic camera the actual numbers here don't thing that much. The objects will appear the same size no affair how far away they are from the camera. What matters, though, is their proportion.
Through this whole tutorial, nosotros saw all the examples through the same camera. This camera was moved by the same unit along every axis and it looks towards the 0,0,0 coordinate. Positioning an orthographic camera is similar positioning a directional calorie-free. It'due south not the bodily position that matters, simply its direction.
How to Return the Scene
So we managed to put together the scene and a camera. Now but the final piece is missing that renders the image into our browser.
We need to define a WebGLRenderer. This is the slice that is capable of rendering the bodily image into an HTML canvas when we provide a scene and a camera. This is also where we tin set the actual size of this canvass – the width and elevation of the sail in pixels every bit it should appear in the browser.
import * as Three from "three"; // Scene const scene = new THREE.Scene(); // Add together a cube to the scene const geometry = new THREE.BoxGeometry(3, 1, 3); // width, summit, depth const fabric = new THREE.MeshLambertMaterial({ colour: 0xfb8e00 }); const mesh = new THREE.Mesh(geometry, material); mesh.position.fix(0, 0, 0); scene.add together(mesh); // Set up lights const ambientLight = new THREE.AmbientLight(0xffffff, 0.vi); scene.add together(ambientLight); const directionalLight = new Iii.DirectionalLight(0xffffff, 0.6); directionalLight.position.set(10, 20, 0); // ten, y, z scene.add(directionalLight); // Camera const width = 10; const height = width * (window.innerHeight / window.innerWidth); const camera = new Three.OrthographicCamera( width / -2, // left width / 2, // right height / 2, // summit height / -ii, // bottom 1, // well-nigh 100 // far ); camera.position.set up(four, 4, iv); camera.lookAt(0, 0, 0); // Renderer const renderer = new Three.WebGLRenderer({ antialias: true }); renderer.setSize(window.innerWidth, window.innerHeight); renderer.render(scene, camera); // Add it to HTML certificate.body.appendChild(renderer.domElement);
And finally, the last line here adds this rendered canvass to our HTML document. And that'due south all you need to render a box. It might seem a little too much for just a unmarried box, but almost of these things we only have to set up upwards once.
If you want to move forward with this project, then bank check out my YouTube video on how to plough this into a simple game. In the video, we create a stack building game. We add game logic, event handlers and blitheness, and even some physics with Cannon.js.
If you have any feedback or questions on this tutorial, experience costless to Tweet me @HunorBorbely or go out a comment on YouTube.
Larn to code for costless. freeCodeCamp'south open source curriculum has helped more than twoscore,000 people get jobs equally developers. Get started
Source: https://www.freecodecamp.org/news/render-3d-objects-in-browser-drawing-a-box-with-threejs/
0 Response to "realistic drawings of 3d objects"
Post a Comment